JavaScript Placement: Head vs. Footer – Pros and Cons
Footer or header JavaScript? What does it matter to the Core Web Vitals and which one should you use?

JavaScript Placement: Head vs. Footer – Pros and Cons
When developing web pages, the placement of JavaScript code can significantly impact the page's performance, loading speed, and user experience. Traditionally, it has been recommended to place JavaScript in the <head> of the page. This is a safe bet, but is it the right choice? In this article, we will explore the differences between JavaScript in the head and in the footer of the page, examining the pros and cons of each approach and the contexts in which they are most suitable.
Background: the preload scanner
JavaScript in the Head:
Placing JavaScript code in the <head> section of the HTML document has some advantages, especially when it comes to code organization and separation from the HTML markup. Here are the pros and cons of using JavaScript in the head:
Pros:
- Code Separation: Keeping JavaScript code in the <head> allows for clear separation between the script logic and the HTML content, making the codebase more maintainable and readable.
- Early Download: JavaScript in the head is queued for download before any of the resources in the body of the page (like image, video's and iframes)
- Earlier execution: Scripts that are enqueued for download are usually executed earlier then scripts in the footer. The exact execution time depends on a lot of factors. If you are interested I suggest you read Defer vs Async JavaScript and how this affects the Core Web Vitals
Cons:
- Render Blocking: When JavaScript is placed in the <head> (without the defer attribute), it can block the rendering of HTML elements, resulting in a delay in the page's display until the script execution is complete.
- Slower Page Load: Due to the early download it competes for other imporact resources like the largest contentful paint element.
When should you place JavaScript in the head?
Important scripts: Scripts that are critical to rendering or critical to the page experience should be placed in the head of the page. For example scripts that handle your menu or your main slider or your cookie notice should be placed in the head of the page.
Feature Detection Scripts: In certain scenarios, JavaScript libraries like Modernizr, which are used for feature detection, may be required in the <head> to detect browser capabilities early in the page's lifecycle.
JavaScript in the Footer:
Placing JavaScript code at the bottom of the page, just before the closing </body> tag, has become a widely used practice. This will enqueue the script for download after the other element in the body like images and video's. This will improve the paint speed over functionality. Here are the pros and cons of using JavaScript in the footer:
Pros:
- Faster Page Load: By placing JavaScript at the end of the page, the HTML content loads first, and the JavaScript executes later, resulting in a faster initial page load.
- Avoiding Single Points of Failure (SPOFs): By loading JavaScript last, the chances of encountering SPOFs are reduced, ensuring that the rest of the page's content is visible even if JavaScript fails to load or execute.
Cons:
- Late Execution: JavaScript in the footer may lead to delayed execution of certain scripts, affecting functionalities that rely on early access to JavaScript.
When should you place JavaScript in the footer?
Less Critical Code: For scripts that do not affect page rendering or functionalities that are not required immediately upon page load, placing them in the footer can lead to better overall performance.
Best Practices and Recommendations:
Considering the different aspects of placing JavaScript in the head and footer, here are some best practices and recommendations:
Render Critical Scripts: Scripts that impact the main content of the page should be loaded render blocking in the head of the page. Try to avoid these types of scripts as they can have a huge impact on the Core Web Vitalsan pagespeed.
Critical Scripts: Scripts that are important to conversion or page interaction should be placed asynced or deferred in the head of the page
Normal Scripts: Unless they impact lay-out or require early access, place regular scripts in the footer to enhance page load speed.
Nice-to-have scripts: Scripts that you could do without if you absolutely had to should be injected once the browser is idle.
Listen to events. The DOMContentLoaded or the load event can ensure that JavaScript executes at or after these timing events regardless of its placement.
The decision to place JavaScript in the head or footer of a web page should be based on the specific requirements of the website and the functionalities of the scripts involved. Placing JavaScript in the head offers code separation and early access benefits but may lead to rendering blockage and slower page loading. Conversely, JavaScript in the footer provides faster page load times and minimizes SPOF risks but can result in delayed execution for certain functionalities. As web developers, it is essential to understand the trade-offs and make informed decisions to optimize the user experience and overall performance of the website.
Example 1: JavaScript in the Head
<!DOCTYPE html>
<html>
<head>
<title>JavaScript in the Head Example</title>
<script>
// JavaScript code in the head
function showMessage() {
alert("Hello from JavaScript in the head!");
}
</script>
<!-- render blocking external scripts -->
<script src="script.js">
In this example, the JavaScript function showMessage()
is placed in the <head>
section of the HTML document. The function is called when the button is clicked, displaying an alert. However, this the body will not be parsed before the file 'script.js' has been loaded and executed.
Example 2: JavaScript in the Footer
<!DOCTYPE html>
<html>
<head>
<title>JavaScript in the Footer Example</title>
</head>
<body>
<!-- NON render blocking external script -->
<script src="script.js"></script>
</body>
</html>
In this example, the same JavaScript function showMessage()
is placed at the bottom of the <body>
section, just before the closing </body>
tag. Placing JavaScript in the footer allows the HTML content to load first, and the JavaScript code will execute when the button is clicked. As a result, the alert appears without any noticeable delay, providing a smoother user experience and this script is not impacted by file 'script.js' since that will be downloaded in the background.
Example 3: Using event Listeners
<!DOCTYPE html>
<html>
<head>
<title>Event listener Example</title>
<script>
// JavaScript event listeners
window.addEventListener('load',function() {
// Code inside this function will execute after the page has loaded
console.log("Page is fully loaded.");
});
</script>
</head>
<body>
<!-- Page content -->
</body>
</html>
In this example, load event listener with callback function is used to ensure that the JavaScript code inside the function is executed only after the page has fully loaded, regardless of whether it is placed in the head or footer. This approach ensures that the code is not executed until all the elements on the page are ready, providing a reliable and consistent behavior.
These examples demonstrate the difference in behavior between JavaScript placed in the head and in the footer. Placing JavaScript in the footer can lead to faster page loading and a smoother user experience, especially when dealing with larger scripts or functionalities that don't require immediate execution. However, certain scenarios, like feature detection or specific library usage, may require JavaScript to be placed in the head. Always consider the specific requirements of your web page and choose the appropriate placement of JavaScript accordingly.
Need your site lightning fast?
Join 500+ sites that now load faster and excel in Core Web Vitals.
- Fast on 1 or 2 sprints.
- 17+ years experience & over 500 fast sites
- Get fast and stay fast!
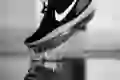
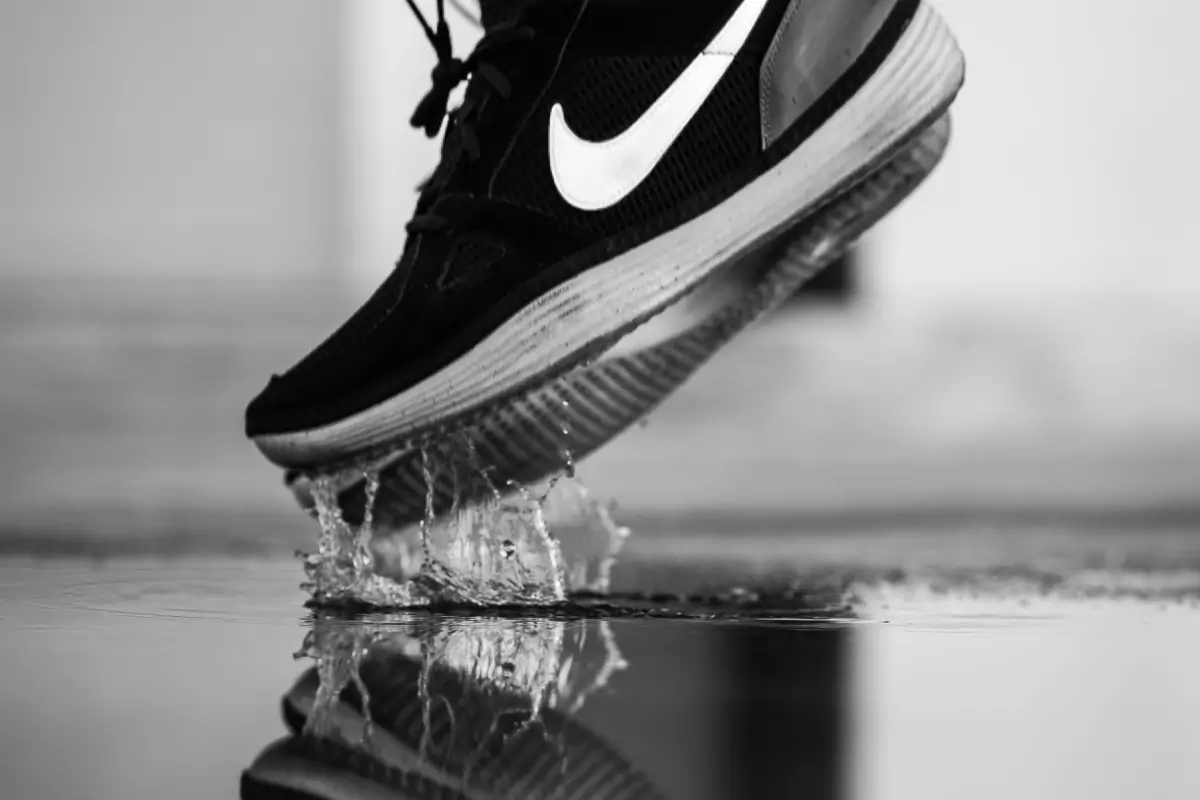